C++에서 변수를 초기화 하는 방법
int a = 123; 같이 초기에 초기화 시키는 방법 copy initialzation
int a(123); 괄호로 초기화 direct initialization
int b { 123 }; 중괄호로 초기화 uniform initialization
밑에 두가지는 직접 데이터 타입을 만들어서 사용할 때 유용하게 사용된다.
강제적으로 데이터 타입을 바꿀때
int i = (int)3.1415 copy initialization
int a((int)3.1415) direct initialization은 타입명을 맞추어서 초기화를 시키면 되지만
uniform initialization은 어떻게든 타입을 맞추어주어야 한다.
-------------
char 문자의 character를 나타내주지만 1바이트여서 최소크기 저장소로 사용되는 경우가 있음.
int i 는 32개의 0 혹은1로 이루어져 있으며 맨 앞 비트는 부호에 사용된다.
unsigned는 부호표현말고 숫자로 사용된다.
#include <iostream>
#include <cmath>
#include <limits>
int main()
{
using namespace std;
short s = 1; // 2 bytes = 2 * 8 bits = 16bits 2^16 숫자 가능
short s1 = 1;
int i = 1;
long l = 1;
long long ll = 1;
cout << sizeof(short) << endl;
cout << sizeof(int) << endl;
cout << sizeof(long) << endl;
cout << sizeof(long long) << endl;
cout << std::pow(2, sizeof(short) * 8 - 1) - 1 << endl;
//표현할 수 있는 가장 큰 숫자를 나타냄
cout << std::numeric_limits<short>::max() << endl;
cout << std::numeric_limits<short>::min() << endl;
cout << std::numeric_limits<short>::lowest() << endl;
//overflow 문제를 주의하라
s = 32767;
s++; //32768를 기대하지만
cout << s << endl; //overflow 숫자를 넘어가면 오히려 뒤로 넘어가게 된다.
s1 = -32768;
s1--;
cout << s1 << endl;
//int, unsigned int도 각각 다 다르다.
return 0;
}
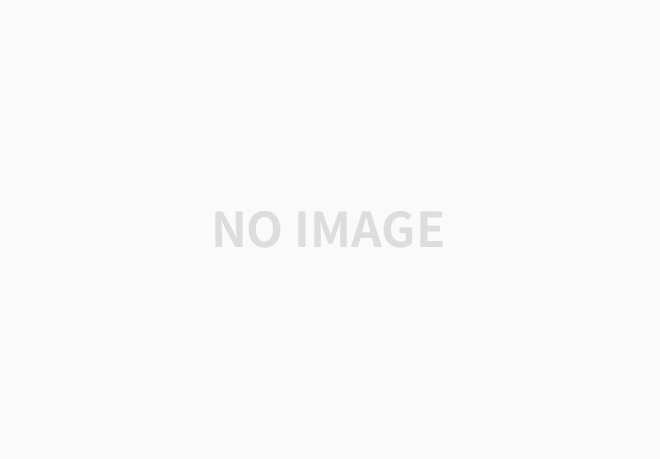
overflow는 숫자 타입의 한계를 넘어갔을 때 의도하지 않은 숫자로 넘어가는 것이다.
문제는 C++은 이러한 현상에 대해서 문제점을 말해주지 않는다. 이진수로 계산이 되기 때문에 이러한 오류를 표기해주지 않고 그대로 진행을 시켜버린다. 그래서 이러한 점에 대해서 잘 확인해 주어야 한다.
여기서 void는 주소 타입을 나타내 주는데 int나 float다 둘다 주소의 타입은 동일하다
--------
float 4 bytes
double
long double
실수 부분에서는
부호 sign - 1 bit | 지수 exponent - 8 bit | 가수 mantissa - 23 bit |
0 | 00001111 | 11000000000000000000000 |
+ | 2^0 + 2^1 + 2^2 = 7 | 2^-1 + 2^-2 = 0.5 + 0.25 = 0.75 |
가수로 표현되기 때문에 뒤에 표현되는 부분하고는 차이점이 있을 수 밖에 없다.
+ (1+ 0.75) x 2^(7-127) = 1.316554 x 10^-36
float type들의 max min 크기 확인 코드
#include <iostream>
#include <iomanip>
#include <limits>
int main()
{
using namespace std;
float f;
double d;
long double ld;
cout << sizeof(float) << endl;
cout << sizeof(d) << endl;
cout << sizeof(ld) << endl;
cout << numeric_limits<float>::max() << endl;
cout << numeric_limits<double>::max() << endl;
cout << numeric_limits<long double>::max() << endl;
cout << numeric_limits<float>::min() << endl;
cout << numeric_limits<double>::min() << endl;
cout << numeric_limits<long double>::min() << endl;
cout << numeric_limits<float>::lowest() << endl;
cout << numeric_limits<double>::lowest() << endl;
cout << numeric_limits<long double>::lowest() << endl;
return 0;
}
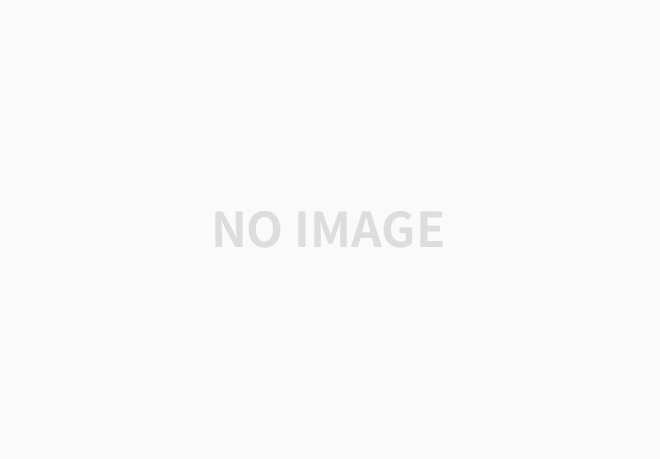
10진수를 이용한 표현법 확인
#include <iostream>
#include <iomanip>
#include <limits>
int main()
{
using namespace std;
float f(3.14); // 3.14 = 31.4 * 0.1
cout << 3.14 << endl;
cout << 31.4e-1 << endl;
cout << 31.4e-2 << endl;
cout << 31.4e1 << endl;
cout << 31.4e2 << endl;
return 0;
}
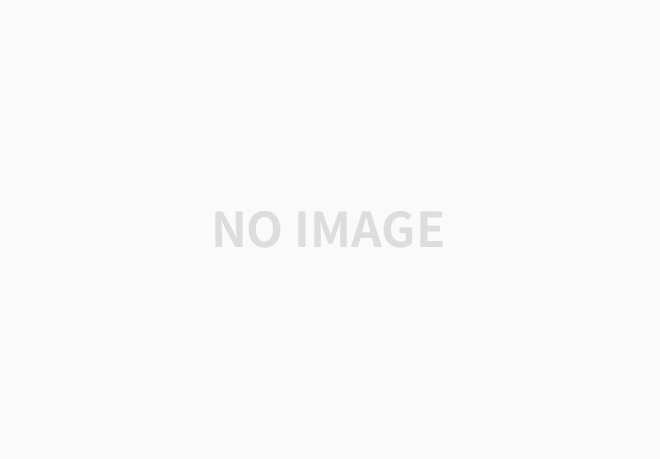
이진수로 저장을 하게 되면 정확도가 조금 떨어질 수도 있다.
#include <iostream>
#include <iomanip>
#include <limits>
int main()
{
using namespace std;
float f(123456789.0f); // 10 significant digits
cout << std::setprecision(16) << endl; //소수점 밑의 갯수를 지정할 수 있음
//123456792로 출력됨. 이진수로 저장하기 때문에 오류가 발생 할 수 있음
cout << f << endl;
// 이러한 오류가 누적되면 잡아내기 어려울 수 있음
return 0;
}
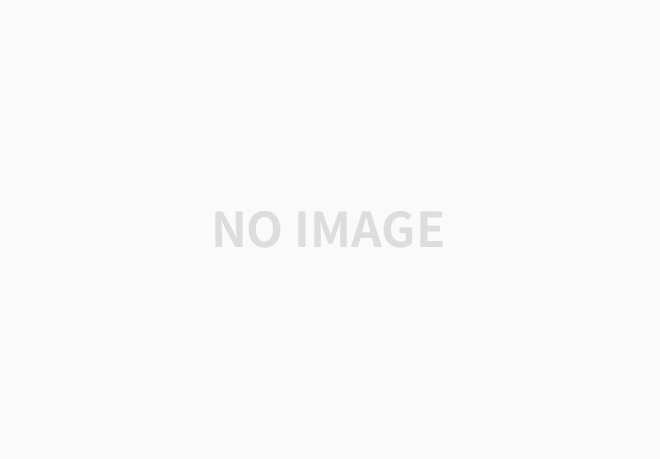
----------
char type
#include <iostream>
#include <limits>
int main()
{
using namespace std;
char c1(65);
char c2('A');
//A A 65 65
cout << c1 << " " << c2 << " " << int(c1) << " " << int(c2) << endl;
// c-style casting
cout << (char)65 << endl;
cout << (int)'A' << endl;
//C++ style casting
cout << char(65) << endl;
cout << int('A') << endl;
cout << c1 << " " << static_cast<int>(c1) << endl;
//char 타입 사이즈 체크
cout << sizeof(char) << endl;
cout << (int)std::numeric_limits<unsigned char>::max() << endl;
cout << (int)std::numeric_limits<unsigned char>::lowest() << endl;
cout << "This is firstl ine \nsecond line \n";
//줄바꿈의 아스키코드
cout << int('\n') << endl;
cout << int('\t') << endl; //tab
return 0;
}
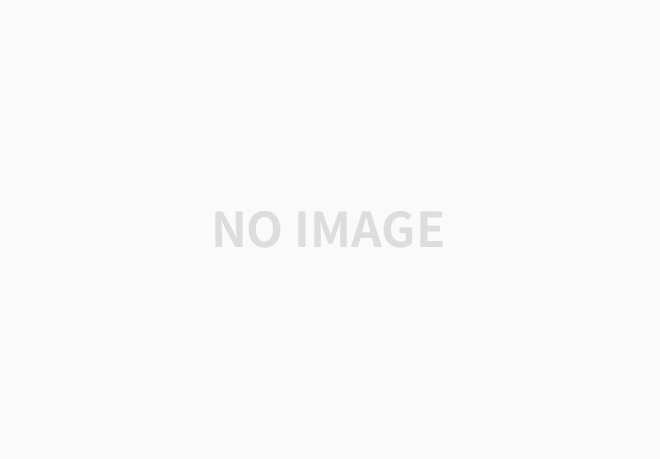
'Programming > C++' 카테고리의 다른 글
C++ 난수 random number 만들기 (0) | 2021.03.14 |
---|---|
C++ 배열의 반복 (0) | 2021.03.14 |
C++ 배열의 기초 (0) | 2021.03.08 |
C++ function (0) | 2021.02.09 |
C++ 공부 (0) | 2021.02.08 |